8.11 Environment actions
8.11.1 Actions for environment
These actions are executed by the environment
actor.
They can be used to specify the environmental conditions for the scenario.
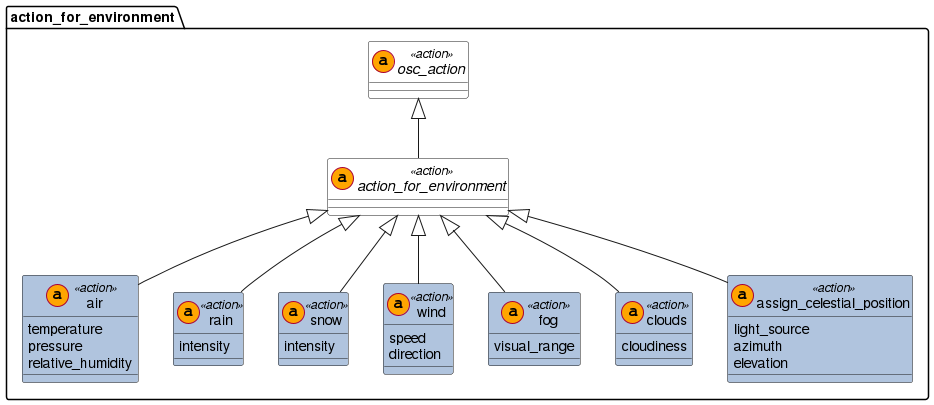
8.11.2 Action air
Specify state changes that relate to the air.
- Basic information
-
Table 159. Basic information of action air Parents
action_for_environment
Used by
Controlled states
States related to air.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 160. Action air Parameter Type Mandatory Description temperature
no
See definition of air struct.
pressure
no
See definition of air struct.
relative_humidity
float
no
See definition of air struct.
- Inherited parameters and variables
-
Table 161. Inherited parameters and variables of action air Parent Inherited parameters and variables
8.11.2.1 Examples
environment.air(temperature: temperature [, pressure: pressure] [, relative_humidity: float] [, <inherited action parameters>])
environment.air(pressure: pressure [, temperature: temperature] [, relative_humidity: float] [, <inherited action parameters>])
environment.air(relative_humidity: float [, temperature: temperature] [, pressure: pressure] [, <inherited action parameters>])
Use one, two or all three action parameters. The unused parameters mean that the action will not modify the corresponding variable.
# All three variables
environment.air(15.0celsius, 1050.0hPa, 0.65)
environment.air(temperature: 15.0celsius, pressure: 1050.0hPa, relative_humidity: 0.65)
# Only temperature and relative humidity (presure is not modified)
environment.air(15.0celsius, relative_humidity: 0.65)
environment.air(temperature: 15.0celsius, relative_humidity: 0.65)
# Only temperature
environment.air(15.0celsius)
environment.air(temperature: 15.0celsius)
# Only pressure
environment.air(pressure: 1050.0hPa)
# Only relative_humidity
environment.air(relative_humidity: 0.65)
8.11.3 Action rain
Specify state changes that relate to rain.
- Basic information
-
Table 162. Basic information of action rain Parents
action_for_environment
Controlled states
States related to rain.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 163. Action rain Parameter Type Mandatory Description intensity
no
See definition of precipitation struct.
- Inherited parameters and variables
-
Table 164. Inherited parameters and variables of action rain Parent Inherited parameters and variables
8.11.4 Action snow
Specify state changes that relate to snow.
- Basic information
-
Table 165. Basic information of action snow Parents
action_for_environment
Controlled states
States related to snow.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 166. Action snow Parameter Type Mandatory Description intensity
no
See definition of precipitation struct.
- Inherited parameters and variables
-
Table 167. Inherited parameters and variables of action snow Parent Inherited parameters and variables
8.11.5 Action wind
Specify state changes related to wind.
- Basic information
-
Table 168. Basic information of action wind Parents
action_for_environment
Used by
Controlled states
States related to wind.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 169. Action wind Parameter Type Mandatory Description speed
no
See definition of wind struct.
direction
no
See definition of wind struct.
- Inherited parameters and variables
-
Table 170. Inherited parameters and variables of action wind Parent Inherited parameters and variables
8.11.5.1 Examples
environment.wind(speed: speed, direction: angle [, <inherited action parameters>])
environment.wind(speed: speed [, <inherited action parameters>])
environment.wind(direction: angle [, <inherited action parameters>])
In the following example, the wind speed should be 3 m/s with an angle of 45 degrees.
# Both variables
environment.wind(3.0mps, 45deg)
environment.wind(speed: 3.0mps, direction: 45deg)
# Only wind speed
environment.wind(3.0mps)
environment.wind(speed: 3.0mps)
# Only wind direction
environment.wind(direction: 45deg)
8.11.6 Action fog
Specify state changes related to fog.
- Basic information
-
Table 171. Basic information of action fog Parents
action_for_environment
Used by
Controlled states
States related to fog.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 172. Action fog Parameter Type Mandatory Description visual_range
no
See definition of fog struct.
- Inherited parameters and variables
-
Table 173. Inherited parameters and variables of action fog Parent Inherited parameters and variables
8.11.7 Action clouds
Specify state changes related to clouds.
- Basic information
-
Table 174. Basic information of action clouds Parents
action_for_environment
Used by
Controlled states
States related to clouds.
Action ending
The action ends when the action of the same type is invoked.
- Parameters
-
Table 175. Action clouds Parameter Type Mandatory Description cloudiness
uint
no
See definition of clouds struct.
- Inherited parameters and variables
-
Table 176. Inherited parameters and variables of action clouds Parent Inherited parameters and variables
8.11.8 Action assign_celestial_position
This action assigns the position of a celestial_light_source such as sun or moon. This will override possibly calculated positions based on geographic location and time. Unless this action is invoked again, the position of the celestial_light_source will remain fixed throughout the scenario.
- Basic information
-
Table 177. Basic information of action assign_celestial_position Parents
action_for_environment
Controlled states
Position of celestial_light_source.
Action ending
The action ends when the position is reached.
- Parameters
-
Table 178. Action assign_celestial_position Parameter Type Mandatory Description light_source
yes
Celestial light source whose position will be assigned.
azimuth
no
See definition of celestial_position_2d struct.
elevation
no
See definition of celestial_position_2d struct.
- Inherited parameters and variables
-
Table 179. Inherited parameters and variables of action assign_celestial_position Parent Inherited parameters and variables
8.11.8.1 Examples
environment.assign_celestial_position(light_source: celestial_light_source, azimuth: angle, elevation: angle
[, <inherited action parameters>])
environment.assign_celestial_position(light_source: celestial_light_source, azimuth: angle
[, <inherited action parameters>])
environment.assign_celestial_position(light_source: celestial_light_source, elevation: angle
[, <inherited action parameters>])
This action assigns the position of a celestial object such as the sun or the moon.
Using the action will override the celestial positions that would be calculated based on geographic location (geodetic_position
) and calendar time (datetime
).
Unless this action is invoked again, the celestial position will remain fixed throughout the scenario.
# For moon position
environment.assign_celestial_position(environment.moon, 270deg, 90deg)
environment.assign_celestial_position(environment.moon, azimuth: 270deg, elevation: 90deg)
# For sun position
environment.assign_celestial_position(environment.sun, 100deg, 40deg)
environment.assign_celestial_position(environment.sun, azimuth: 100deg, elevation: 40deg)
# For sun, assign only azimuth
environment.assign_celestial_position(environment.sun, azimuth: 100deg)
# For sun, assign only elevation
environment.assign_celestial_position(environment.sun, elevation: 40deg)