8.7 Physical object actors
8.7.2 Actor physical_object
All tangible objects close to earth, excluding celestial objects such as sun or moon.
- Basic information
-
Table 13. Basic information of actor physical_object Instantiable
no
Parents
osc_actor
Children
- Parameters
-
Table 14. Actor physical_object Parameter Type Mandatory Description yes
See bounding_box
no
See color
geometry_reference
string
no
Opaque reference of an associated 3D geometry model of the physical object. It is implementation-specific how model references are resolved to 3D models.
center_of_gravity
yes
Center of gravity of the object. If unknown, the center of the bounding box may be used instead.
- State variables
-
Table 15. State variables of actor physical_object Variable Type Mandatory Description pose
yes
Position and orientation measured in world coordinates with world system as reference.
8.7.2.1 Methods
8.7.2.1.1 Method object_distance()
Returns the relative distance between the physical_object
that calls the method and a reference entity.
The distance is computed as the position of the reference
entity, measured in the coordinate system of the physical_object
that calls the method.
This calculation is performed either in the longitudinal direction (x-axis) or the lateral direction (y-axis).
By default, the distance is measured between the reference points of the respective entities. Optionally, the distance between the bounding boxes can be computed instead.
- Prototype
-
extend physical_object: def object_distance(reference: physical_object, direction: distance_direction, mode: distance_mode) -> length
- Return value
-
The relative distance between the
physical_object
that calls the method and the reference entity. - Parameters
-
Table 16. Parameters for method object_distance() Parameter Type Description reference
The reference entity.
direction
-
Use
longitudinal
to measure the distance in the x-coordinate. -
Use
lateral
to measure the distance in the y-coordinate.
mode
-
Use
reference_points
to measure the distance between the reference points. (Default) -
Use
bounding_boxes
to measure the distance between the bounding boxes.
-
8.7.2.1.2 Method get_s_coord()
Returns the s-coordinate of the physical_object
.
By default, the method uses the s-axis of the road where the physical_object
is located, but the user can select other route element types.
If the physical_object
is not on a route the method returns an error.
- Prototype
-
extend physical_object: def get_s_coord(route_type: on_route_type) -> length
- Return value
-
Returns a length with the s-coordinate.
- Parameters
-
Table 17. Parameters for method get_s_coord() Parameter Type Description route_type
Select the type of route that will be used to compute the s-coordinate:
-
on_road
(default)
Use the s-axis of theroad
where the actor is located. If the actor is not on a road the method returns an error. -
on_lane_section
Use the s-axis of thelane_section
where the actor is located. If the actor is not on a lane section the method returns an error. -
on_lane
Use the s-axis of thelane
where the actor is located. If the actor is not on a lane the method returns an error. -
on_crossing
Use the s-axis of thecrossing
where the actor is located. If the actor is not on a crossing the method returns an error.
-
8.7.2.1.3 Method get_t_coord()
Returns the t-coordinate of the physical_object
.
By default, the method uses the t-axis of the road where the physical_object
is located, but the user can select other route element types.
If the physical_object
is not on a route the method returns an error.
- Prototype
-
extend physical_object: def get_t_coord(route_type: on_route_type) -> length
- Return value
-
Returns a length with the t-coordinate.
- Parameters
-
Table 18. Parameters for method get_t_coord() Parameter Type Description route_type
Select the type of route that will be used to compute the t-coordinate:
-
on_road
(default)
Use the t-axis of theroad
where the actor is located. If the actor is not on a road the method returns an error. -
on_lane_section
Use the t-axis of thelane_section
where the actor is located. If the actor is not on a lane section the method returns an error. -
on_lane
Use the t-axis of thelane
where the actor is located. If the actor is not on a lane the method returns an error. -
on_crossing
Use the t-axis of thecrossing
where the actor is located. If the actor is not on a crossing the method returns an error.
-
8.7.2.1.4 Method get_route_point()
Returns the route_point
where the physical_object
is located.
By default, the method uses the road where the physical_object
is located, but the user can select other route element types.
If the physical_object
is not on a route the method returns an error.
- Prototype
-
extend physical_object: def get_route_point(route_type: on_route_type) -> route_point
- Return value
-
Returns a
route_point
. - Parameters
-
Table 19. Parameters for method get_route_point() Parameter Type Description route_type
Select the type of route that will be used to compute the route_point:
-
on_road
(default)
Use the t-axis of theroad
where the actor is located. If the actor is not on a road the method returns an error. -
on_lane_section
Use the t-axis of thelane_section
where the actor is located. If the actor is not on a lane section the method returns an error. -
on_lane
Use the t-axis of thelane
where the actor is located. If the actor is not on a lane the method returns an error. -
on_crossing
Use the t-axis of thecrossing
where the actor is located. If the actor is not on a crossing the method returns an error.
-
8.7.2.2 Examples
# Constrain vehicle to be a wide car
my_wide_car: vehicle
keep(my_wide_car.vehicle_category == car)
keep(my_wide_car.bounding_box.width >= 1.95m)
keep(my_wide_car.color == maroon)
keep(my_wide_car.axles.size() == 2)
keep(my_wide_car.axles[0].number_of_wheels == 2)
keep(my_wide_car.axles[1].number_of_wheels == 2)
keep(my_wide_car.intended_infrastructure[0] == driving)
# Constrain vehicle to be a motorcycle
my_motorcycle: vehicle
keep(my_motorcycle.vehicle_category == vru_vehicle)
keep(my_motorcycle.axles.size() == 2)
keep(my_motorcycle.axles[0].number_of_wheels == 1)
keep(my_motorcycle.axles[1].number_of_wheels == 1)
keep(my_motorcycle.intended_infrastructure[0] == driving)
# Constrain vehicle to be a bicycle
my_bicycle: vehicle
keep(my_bicycle.vehicle_category == vru_vehicle)
keep(my_bicycle.axles.size() == 2)
keep(my_bicycle.axles[0].number_of_wheels == 1)
keep(my_bicycle.axles[1].number_of_wheels == 1)
keep(my_bicycle.intended_infrastructure[0] == biking)
8.7.3 Actor stationary_object
A stationary_object is a physical_object that is anchored and whose bounding box cannot change its position or speed like a tree or a building.
- Basic information
-
Table 20. Basic information of actor stationary_object Instantiable
yes
Parents
- Inherited parameters and variables
-
Table 21. Inherited parameters and variables of actor stationary_object Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.3.1 Modifiers
8.7.3.1.1 Modifier location()
Specifies the location of a stationary_object
.
Shall be invoked before the do
section, as the location cannot be time-dependent.
- Parameters
-
Table 22. Parameters for modifier stationary_object.location() Parameter Type Description pose
Location of the stationary object for the whole scenario.
- Syntax
-
Code 2. Syntax examples for stationary_object.location()
my_pose: pose_3d # Add constraints for fields of my_pose my_building: stationary_object my_building.location(my_pose) do: ...
8.7.4 Actor movable_object
A movable_object is a physical_object that is not anchored and can, therefore, be moved and change its position and speed like a rock or a ball.
- Basic information
-
Table 23. Basic information of actor movable_object Instantiable
yes
Parents
Children
- State variables
-
Table 24. State variables of actor movable_object Variable Type Mandatory Description velocity
yes
Translational and rotational velocity measured in object coordinates with world system as reference.
acceleration
yes
Translational and rotational acceleration measured in object coordinates with world system as reference.
speed
yes
Speed in center_of_gravity defined as sqrt(velocity.translational.x² + velocity.translational.y²) * sign(velocity.translational.x)
- Inherited parameters and variables
-
Table 25. Inherited parameters and variables of actor movable_object Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.4.1 Methods
8.7.4.1.1 Method distance_along_route()
Returns the distance between an actor and the start or end of a route, measured along the s-axis of the route. User can pass either a route_element or a compound_route.
- Prototype
-
extend traffic_participant: def distance_along_route(route: route, from: route_distance_enum) -> length
- Return value
-
Returns a length measured along the s-axis of the route.
- Parameters
-
Table 26. Parameters for method distance_along_route() Parameter Type Description route
The route along which the distance should be measured.
If the actor calling the method is not on the given route the method returns an error.from
Reference point from which the distance is measured.
-
from_start
(default)
Method returns the relative s-coordinate from route.start_point() to the actor.
Positive means that the traffic participant is ahead of the start point. -
from_end
Method returns the relative s-coordinate from the actor to the route.end_point().
Positive means that the traffic participant is behind of the end point.
-
8.7.5 Actor traffic_participant
A traffic_participant is a physical_object that is relevant within traffic and may perform traffic-related actions (see traffic_participant actions) such as following a route. A traffic_participant includes both, objects that do participate in traffic actively like a driven vehicle and objects that do not move throughout the scenario like a parked vehicle.
- Basic information
-
Table 27. Basic information of actor traffic_participant Instantiable
no
Parents
Children
- Parameters
-
Table 28. Actor traffic_participant Parameter Type Mandatory Description list of intended_infrastructure
yes
See intended_infrastructure for definition. Intended usage is for further specification of an entity. For example, together with vehicle_category or to provide hints for implemenations where to spawn and / or auto-route entities. Note that multiple types of infrastructure can be assigned because of the list character of this type.
- Inherited parameters and variables
-
Table 29. Inherited parameters and variables of actor traffic_participant Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.5.1 Methods
8.7.5.1.1 Method time_to_collision()
Returns the time that is left until a possible collision between a traffic_participant and a reference physical_object takes place.
- Prototype
-
extend traffic_participant: def time_to_collision(reference: physical_object) -> time
- Return value
-
Returns the time until the bounding_box of traffic_participant would collide with the bounding box of the reference physical_object, assuming both keep moving with their current velocity.
- Parameters
-
Table 30. Parameter for method time_to_collision() Parameter Type Description reference
The reference physical_object.
- Syntax
-
Code 3. Syntax example for method time_to_collision()
scenario prevent_collision: ego: vehicle other_car: vehicle var collision_time: time = sample(ego.time_to_collision(reference: other_car), every(2s)) do parallel: other_car.drive() with: speed(50kph) ego.drive() with: position(behind: other_car) until rise(collision_time < 10s)
8.7.5.1.2 Method time_headway()
Returns the time distance between a traffic participant and a reference physical_object.
- Prototype
-
extend vehicle: def time_headway(reference: physical_object) -> time
- Return value
-
Returns the time distance between traffic participant and reference physical_object, measured from reference point to reference point along s-coordinate, assuming the reference physical_object keeps moving with its current speed.
- Parameters
-
Table 31. Parameter for method time_headway() Parameter Type Description reference
The reference physical_object.
8.7.5.1.3 Method space_gap()
Returns the space distance between a traffic participant and a reference physical_object.
- Prototype
-
extend traffic_participant: def space_gap(reference: physical_object, direction: distance_direction) -> length
- Return value
-
Returns the space distance between the bounding_box of the traffic_participant that calls the method and the reference physical_object.
- Parameters
-
Table 32. Parameters for method space_gap() Parameter Type Description reference
The reference entity.
direction
-
longitudinal
The relative s-coordinate.
Positive means that the traffic participant is ahead of the reference. -
lateral
The relative t-coordinate.
Positive means that the traffic participant is left of the reference.
-
8.7.6 Actor vehicle
A device intended for transport of people or cargo. Here restricted to land vehicles, meaning vehicles that move on a land surface such as a road or sidewalk.
- Basic information
-
Table 33. Basic information of actor vehicle Instantiable
yes
Parents
- Parameters
-
Table 34. Actor vehicle Parameter Type Mandatory Description yes
See vehicle_category
axles
list of axle
yes
See axle
rear_overhang
yes
Rear overhang of the vehicle or more explicitly the horizontal distance between the end of the bounding_box and the center of the rear axle.
- Inherited parameters and variables
-
Table 35. Inherited parameters and variables of actor vehicle Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.7 Actor person
A person represents a human being. A person may act in different and changing modalitites throughout a scenario. For example, walking or being in a car.
- Basic information
-
Table 36. Basic information of actor person Instantiable
yes
Parents
- Inherited parameters and variables
-
Table 37. Inherited parameters and variables of actor person Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.8 Actor animal
An animal represents a living being which is not a human. An animal may act in different and changing modalitites throughout a scenario. For example, running or being in a car.
- Basic information
-
Table 38. Basic information of actor animal Instantiable
yes
Parents
- Inherited parameters and variables
-
Table 39. Inherited parameters and variables of actor animal Parent Inherited parameters and variables pose, bounding_box, color, geometry_reference, center_of_gravity
8.7.9 Struct bounding_box
Simplified three dimensional shape enclosing the physical_object. The bounding box does NOT include side mirrors for vehicles. The height includes the ground_clearance, therefore it always goes from the top to the ground.
- Basic information
-
Table 40. Basic information of struct bounding_box Instantiable
no
- Parameters
-
Table 41. Struct bounding_box Parameter Type Mandatory Description center
yes
Represents the geometrical center of the bounding box expressed in coordinates that refer to the coordinate system of the physical_object
length
yes
Dimension in x-direction of the coordinate system of the physical_object
width
yes
Dimension in y-direction of the coordinate system of the physical_object
height
yes
Dimension in z-direction of the coordinate system of the physical_object
8.7.10 Struct axle
Taken from ASAM OpenSCENARIO 1.x plus number of wheels to avoid ambiguities (for example, twin tires). Check definitions there for now.
- Basic information
-
Table 42. Basic information of struct axle Instantiable
no
Used by
- Parameters
-
Table 43. Struct axle Parameter Type Mandatory Description max_steering
yes
Maximum steering angle for the wheels on the axle
wheel_diameter
yes
Diameter for the wheels on this axle
track_width
yes
Distance between the centerline of the outer wheels on opposing sides of the axle
position_x
yes
Longitudinal position of the axle in the vehicle ' s x-axis. For a 2-axle vehicle, the rear axle must have position_x = 0m
position_z
yes
number_of_wheels
uint
yes
Number of wheels on the axle
8.7.11 Enum color
Description of the color of a physical_object. Specifies the color of a physical object. Not intended to replace more detailed material properties, but rather for debugging purposes. For a vehicle this should affect the vehicle body, for pedestrians it may affect the main piece of clothing. Based on the set defined by W3C for HTML_ "basic colors".
- Basic information
-
Table 44. Basic information of enum color Instantiable
no
- Values
-
Table 45. Enum color Value Comment white
RGB(255,255,255)
silver
RGB(192,192,192)
gray
RGB(128,128,128)
black
RGB(0,0,0)
red
RGB(255,0,0)
maroon
RGB(128,0,0)
yellow
RGB(255,255,0)
olive
RGB(128,128,0)
lime
RGB(0,255,0)
green
RGB(0,128,0)
aqua
RGB(0,255,255)
teal
RGB(0,128,128)
blue
RGB(0,0,255)
navy
RGB(0,0,128)
fuchsia
RGB(255,255,0)
purple
RGB(255,0,255)
8.7.12 Enum vehicle_category
Vehicle category based on UN ECE/TRANS/WP.29/78/Rev.6 extended by non-self-propelled vehicles.
- Basic information
-
Table 46. Basic information of enum vehicle_category Instantiable
no
Used by
- Values
-
Table 47. Enum vehicle_category Value Comment car
Power-driven vehicle with maximum mass not exceeding 3.5 t having at least four wheels comprising not more than eight seats in addition to the driver seat. Designed for the carriage of passengers or small goods. (UN category L7,M1,N1)
bus
Power-driven vehicle having at least four wheels comprising more than eight seats in addition to the driver seat.(UN category M2,M3)
truck
Power-driven vehicle with maximum mass exceeding 3.5t having at least four wheels. Designed for the carriage of goods. (UN category N2,N3)
trailer
Vehicle designed to be towed by another vehicle. Non-permanently connected to towing vehicle, meaning it can be separated by an operation without involving facilities normally only found in workshop.
vru_vehicle
Vehicle without a crash-resistant passenger cell intended for one to few passengers or small goods transport. With its occupant it results in a vulnerable road user. (UN category L1-L6 plus bicycles, pedelecs, e-bicycles, personal mobility devices, wheelchairs, mobility scooters and so on.)
other
Unspecified but known type of vehicle (for example, stroller, shopping cart, …)
8.7.13 Enum intended_infrastructure
Infrastructure that is typically used by a traffic participant. Can be used to further specify an entity. For example, together with vehicle_category or to provide hints for simulators where to spawn entities. Each entity can be assigned multiple values. Note that this needs to be aligned with road abstraction feature.
- Basic information
-
Table 48. Basic information of enum intended_infrastructure Instantiable
no
Used by
- Values
-
Table 49. Enum intended_infrastructure Value Comment driving
taken from ASAM OpenDRIVE
sidewalk
A lane meant for people to walk on. Taken from ASAM OpenDRIVE
biking
taken from ASAM OpenDRIVE
rail
taken from ASAM OpenDRIVE
tram
taken from ASAM OpenDRIVE
bus
taken from ASAM OpenDRIVE
taxi
taken from ASAM OpenDRIVE
hov
taken from ASAM OpenDRIVE
8.7.14 Enum distance_direction
- Basic information
-
Table 50. Basic information of enum distance_direction Used by
- Values
-
Table 51. Enum distance_direction Value Comment longitudinal
Measure distance in the x-coordinate. Positive means that the
reference
is in front of thephysical_object
that calls the method.lateral
Measure distance in the y-coordinate. Positive means that the
reference
is to the left of thephysical_object
that calls the method.
8.7.15 Enum distance_mode
- Values
-
Table 52. Enum distance_mode Value Comment reference_points
Measure the distance between the reference points.
bounding_boxes
Measure the distance between the bounding boxes.
8.7.16 Enum on_route_type
Select which s/t coordinate system is used to obtain the coordinates
- Values
-
Table 53. Enum on_route_type Value Comment on_road
Use the
road
s/t coordinateson_lane_section
Use the
lane_section
s/t coordinateson_lane
Use the
lane
s/t coordinateson_crossing
Use the
crossing
s/t coordinates
8.7.17 Enum route_distance_enum
Reference point from which the distance is measured.
- Values
-
Table 54. Enum route_distance_enum Value Comment from_start
Measure distance from the start of the route.
from_end
Measure distance from the end of the route.
8.7.18 Groups of traffic participants
Please note that the following section and its sub-sections are non-normative. |
Many implementations for the creation and the handling of groups of traffic participants exist. Some traffic flow simulators use stochastic traffic flow models and others use deterministic modeling approaches.
The following content is an example on how to build groups using only generic ASAM OpenSCENARIO language features. A standard group model may be included in future versions of the standard.
Groups represent multiple actor instances with multiple definitions and states.
It is assumed that scenario authors will likely define groups at the top level and not under lower levels in the domain model hierarchy such as physical_actor .
|
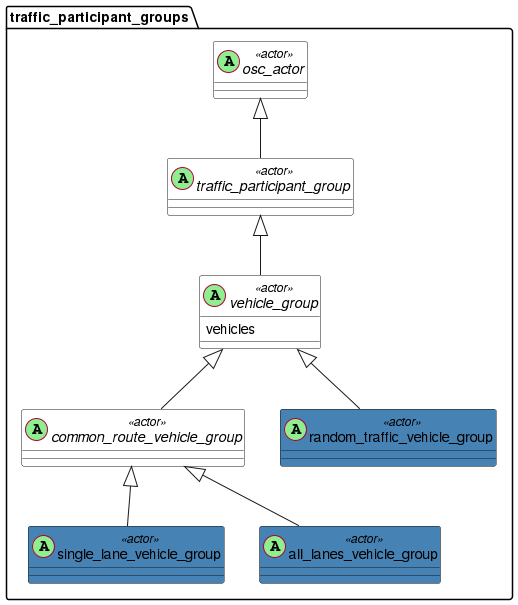
8.7.18.1 Actor vehicle_group
A traffic_participant_group serves as a collection of traffic_participant actors. A group represents multiple actor instances with multiple definitions and states.
There are two main use cases for vehicle groups:
-
Adding entities to a scenario to have more participants in the scenario. By generating multiple vehicles in the surrounding environment of the scenario these vehicles act as 'noise' in the scenario.
-
Creating multiple vehicles with predefined initial conditions.
For example, creating a single-lane or convoy formation of vehicles, or both.
Initial conditions of actors and the actual behavior of actors throughout the scenarios differ:
-
The group defines the initial conditions of actors in the scenario, meaning their creation and destruction.
-
The behavioral model assigned to each vehicle defines the actual behavior of the vehicle within the group.
- Basic information
-
Table 55. Basic information of actor vehicle_group Instantiable
no
Parents
traffic_participant_group
Children
common_route_vehicle_group, random_traffic_vehicle_group
- Parameters
-
Table 56. Actor vehicle_group Parameter Type Mandatory Description vehicles
list of vehicle
yes
List of vehicles being part of this group.
8.7.18.1.1 Examples
scenario dut.driving_alongside_group:
# Defining a group with a common route and
# a single-lane formation
g: single_lane_vehicle_group
# Using 'for' constraint to control vehicles
# parameters
for v in g.vehicles:
keep(v.category in [bus, truck])
r: route
do parallel:
g.drive() with:
along(r)
lane(side_of: dut.vehicle, at: start)
dut.vehicle.drive() with:
along(r)